Table of Contents
Introduction
Understanding and responding to user preferences is a significant aspect in today's technology-driven world. Companies such as Netflix, Amazon, and YouTube use recommendation engines to enhance user experience, provide personalized content, and ultimately increase their customer engagement. At the heart of these recommendation engines is machine learning and Python is one of the most frequently used languages for this due to its simplicity and extensive collection of libraries.
This article provides a comprehensive guide on how to build recommendation engines using Python, offering actionable insights for Python developers, data scientists, analysts, students, and researchers interested in this field. We will discuss the fundamental concepts around recommendation systems such as collaborative filtering and content-based filtering as well as the different machine learning algorithms used in these systems. We will also dive into the practical implementation using Python, exploring popular libraries such as Surprise, LensKit, and LightFM among others.
The concepts and processes explained here are not only applicable to digital media platforms but also span various other domains. For instance, an e-commerce platform can provide product suggestions based on users' past purchases or browsing history, a music application can recommend songs aligned with users' tastes, or a news portal can suggest articles catering to users' reading habits.
Whether you are a Python developer planning to broaden your knowledge, a data scientist aiming to leverage recommendation engines in your data analysis work, or a student or researcher seeking to understand and implement recommendation systems in your projects or studies, this comprehensive guide will be your valuable resource. Join us as we explore the techniques and tools involved in creating recommendation engines using Python, and learn how to make systems that can accurately anticipate users' preferences and enhance their overall experience.
Understanding recommendation engines: An overview
Recommendation engines, also known as recommender systems, are a class of machine learning algorithms that play a crucial role in personalized content suggestion. They analyze users' behavior and preferences to predict and rank items or services a user might be interested in. These predictions are made based on various factors, including users' past activities, search history, and demographic information.
There are three main types of recommendation systems:
1. Content-Based Filtering: This approach focuses on the properties of items. Using item features such as author, genre, or director in the context of a movie recommendation system, content-based filtering systems suggest items that are similar to items a user has liked in the past. The assumption here is simply that if a user has liked a certain type of item in the past, they are likely to like such items in the future as well.
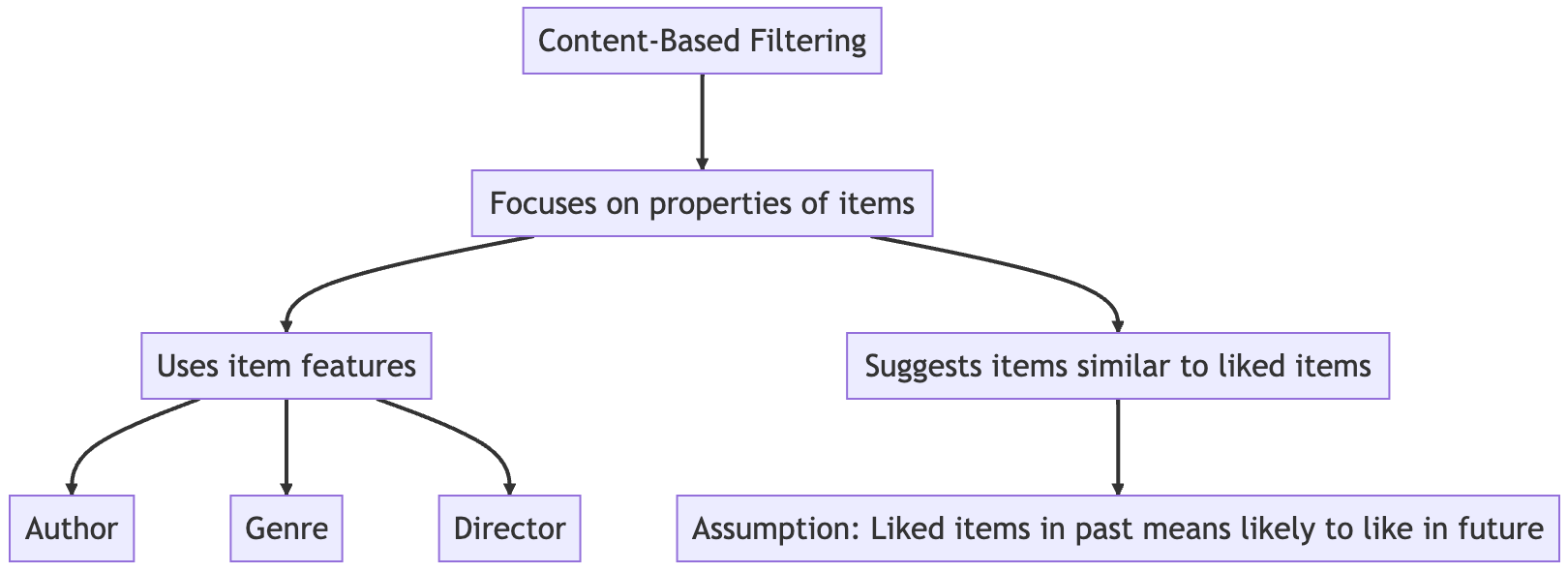
2. Collaborative Filtering: Collaborative filtering uses the behavior of multiple users to recommend items. It operates under the assumption that if two users agreed in the past, they will likely agree in the future. There are two types of collaborative filtering. User-User Collaborative Filtering, which finds users who are similar to the target user based on similarity of ratings, and recommends items that those similar users liked. And, Item-Item Collaborative Filtering, which instead of taking a user-based approach, takes an item-based perspective and recommends items that are similar based on users' interactions with them.

3. Hybrid Systems: These systems combine the strengths of the above two approaches. They might use collaborative filtering to find users who are similar, and then within that user subset, use content-based filtering to find the most suitable items to recommend.
Additionally, while not a distinct type, it's worth noting that Deep Learning-based recommendation systems have also gained significant popularity. They use neural networks to learn from vast amounts of data and make predictions in a way that is harder for traditional systems to accomplish.
All these recommendation engines have their own strengths and weaknesses and are applied according to the needs of the specific application. Some systems might even use combinations of these methods to overcome the limitations of a single approach. Python provides multiple libraries and tools to help developers build, analyze, and deploy these systems efficiently.
Gotcha
Despite their potential, recommendation engines aren't a panacea. Challenges such as the 'cold start' problem - when there is insufficient data about new users or items for the system to provide reliable recommendations - need to be addressed. We will investigate some strategies to overcome this later.
Nonetheless, the power of recommendation engines to improve user experience and promote customer engagement makes them a valuable asset in a wide range of industries.
Exploring the types of recommendation systems: Collaborative filtering and content-based filtering
Digging deeper into the types of recommendation systems, let's unpack the details of two fundamental approaches - collaborative filtering and content-based filtering - which are commonly implemented in Python.
Collaborative Filtering
Collaborative filtering works on the principle of user behavior similarity - 'Users who agreed in the past will agree in the future'. In other words, if two users had similar tastes in the past, it is highly probable they will have similar preferences in the future as well. Collaborative filtering comes in two flavors:
1. User-Based Collaborative Filtering: Also known as user-user collaborative filtering, this approach looks at user behavior and preferences. It analyzes past user-product interactions and assumes that similar users have similar preferences. This method finds users who are similar to the target user based on similarity of ratings, and recommends items that these similar users liked.
2. Item-Based Collaborative Filtering: This method is a bit different in that it proposes recommendations based upon the similarity between items, not users. It considers the set of items that a user has rated, and calculates the likeness between these items and the target item. The items that are deemed most similar are then recommended to the user.
User-item interaction data, such as ratings, is typically stored in a matrix known as the utility matrix. Despite its simplicity, collaborative filtering can be quite effective.
Content-Based Filtering
Content-based filtering, on the other hand, focuses on the properties or features of items. It recommends items by comparing the content of the items and a user profile. For instance, if a user has shown interest in a specific genre of movies, the system will recommend other movies from the same genre.
To create an item profile, properties such as actors, directors, and genres are considered. For users, a profile is created using a utility matrix that describes the relationship between users and items. The system then compares these profiles to generate recommendations.
Though content-based filtering systems offer more personalized recommendations, they may limit the diversity of the recommendations as they are solely based on user's past behavior.
In the next section, we will discuss how Python, with its rich ecosystem of libraries and packages, can be used to implement these types of recommendation systems, walking you through some practical code examples.
Introduction to Machine Learning Algorithms for Recommendation Engines
Machine Learning algorithms form the backbone of recommendation engines. They facilitate the task of analyzing massive datasets, identifying patterns and correlations, and ultimately enabling the system to learn from past user behavior and interactions to make accurate predictions and recommendations. The choice of algorithm depends on the type of recommendation approach used: collaborative filtering, content-based filtering, or a hybrid of the two.
Let's explore some of these algorithms commonly used in recommendation systems:
1. Matrix Factorization: This is an extensively used technique in recommendation systems, specifically in collaborative filtering. Matrix factorization algorithms work by 'decomposing' the user-item interaction matrix into the product of two lower dimensional matrices. This way, they can capture the underlying factors or features that led to a particular user-item interaction. One popular algorithm that uses matrix factorization is Singular Value Decomposition (SVD). Implementing this with Python’s Surprise package is a common approach to build recommendation systems based on rating data.
2. Association Rules Learning: This method is based on the concept of 'if this, then that', providing insights into the associations and correlations between different item sets. The Apriori algorithm, which is used to learn these association rules, has been applied in recommendation systems. This algorithm can be used to find items that get bought together frequently, and then suggest them to users who have bought one of those items.
3. Cosine Similarity: Typically used in content-based filtering, cosine similarity compares item features to generate a similarity score, which is used for recommendations. This technique measures the cosine of the angle between two vectors in a multidimensional space. These vectors can represent items or users, and the similarity score can indicate how alike two items/users are based on their characteristics or behavior.
4. Collaborative Filtering Algorithms: Memory-Based Collaborative Filtering uses user behavior for recommendations and includes algorithms like user-user and item-item collaborative filtering. On the other hand, Model-Based Collaborative Filtering uses machine learning models to predict user ratings for unrated items. This category includes algorithms like K-Nearest Neighbors (KNN), which finds the most similar items/users to make recommendations, and Neural networks and Deep learning based models that can handle vast amounts of data and complex features.
5. Content-Based Algorithms: These algorithms use item features to recommend other items similar to what the user has liked in the past. Techniques like TF-IDF (Term Frequency-Inverse Document Frequency) can be used to convert text into numerical data, and then cosine similarity or other similarity measures can be used to find the most similar items.
It's worth noting that the above list is not exhaustive. There are many other machine learning algorithms and methods used in recommendation systems, and the choice largely depends on the nature of the problem, the available data, and the desired outcome. Despite the variety, Python offers numerous libraries and tools that make it easier to implement these algorithms effectively and efficiently. In the following sections, we will delve into some of these Python resources that can aid in building robust recommendation systems.
Getting started with Python libraries for recommendation systems
Python, with its rich ecosystem of libraries and packages, lends itself perfectly to building recommendation systems. These libraries provide essential tools and algorithms that significantly simplify the process of developing, deploying, and evaluating recommendation models. Let's take a look at some of these libraries and how they can be used in the context of recommendation engines:
1. Surprise (Scikit-learn for recommender systems): Surprise is a Python library that provides various ready-to-use prediction algorithms such as singular value decomposition (SVD), K-Nearest Neighbours (KNN), among others. It also provides tools for evaluating, analyzing, and comparing the performance of different algorithms. The simplicity of its API makes it a popular choice among both beginners and experienced developers.
2. LightFM: LightFM is another powerful Python library for building recommendation systems. It stands out by supporting both collaborative and content-based filtering, allowing for the creation of hybrid models. The library is designed to handle both explicit and implicit feedback, making it highly adaptable to different types of recommendation scenarios.
3. LensKit: LensKit is a set of tools and libraries that make it easier to experiment with recommendation algorithms. It provides functionalities for numerous collaborative and content-based filtering algorithms, along with tools for evaluation and analysis. Its modular design allows for easy extension and customization of existing algorithms.
4. fastFM: fastFM is a library that offers efficient implementation of factorization machines, a class of models that is highly effective for recommendation systems. fastFM provides functionality for regression, binary classification, and ranking tasks. Its ability to handle high-dimensional sparse data makes it a powerful tool when working with large datasets.
5. TensorRec: TensorRec is a recommendation framework built in Python with the power of TensorFlow. It allows developers to create complex recommendation models without having to write a lot of custom code. TensorRec's flexible framework can accommodate both collaborative and content-based models, and can even implement hybrid models.
6. Rexy: Rexy is an open-source recommendation engine which simplifies the development of both collaborative filtering and content-based recommendation systems. It provides a straightforward API and a robust set of features, including support for custom recommendation algorithms.
When selecting a library for your recommendation engine project, consider factors such as the nature of your data, the type of recommendation system you are building, and the level of customization you require. If you need to handle complex scenarios or require more control over your model, a more flexible library like TensorRec or fastFM may be suitable. Conversely, if you are looking for simplicity and ease-of-use, libraries like Surprise or LightFM could be the better choice.
Regardless of the library you choose, remember that the goal is to develop a recommendation system that successfully enhances user experience by providing personalized and relevant recommendations. The power of Python libraries coupled with the right approach can help you create robust and efficient recommendation systems that cater to your unique requirements.
Building a basic recommendation engine with Python: A step-by-step guide
Building a recommendation engine may seem like a daunting task, but Python, with its rich set of libraries, simplifies the process and breaks it down into digestible chunks. Let's build a simple recommendation system using Python. For this step-by-step tutorial, we'll be using the `Surprise` library, which provides tools and algorithms for building recommendation systems.
Our recommendation engine will be based on collaborative filtering and will use the Singular Value Decomposition (SVD) algorithm, a popular choice for recommendation systems.
Before we dive in, make sure that you have the `surprise` library installed in your Python environment. If not, you can install it using pip.
pip install scikit-surprise
Step 1: Import the necessary libraries
The first step is to import the necessary libraries.
from surprise import SVD
from surprise import Dataset
from surprise.model_selection import cross_validate
Step 2: Load the dataset
Next, we'll load our dataset. `Surprise` provides a few built-in datasets. For our exercise, we're using the widely used MovieLens dataset.
data = Dataset.load_builtin('ml-100k')
Step 3: Define the algorithm
Now, we need to define which algorithm we'll use. As mentioned, we're going to use the `SVD` algorithm.
algo = SVD()
Step 4: Fit and evaluate the model
We're now ready to fit and evaluate our model. We'll use 5-fold cross-validation, which means the `cross_validate` function will split the dataset into 5 parts, train the model on 4 parts, and evaluate it on the remaining part. This process is repeated 5 times so that every part of the dataset is used for evaluation.
cross_validate(algo, data, measures=['RMSE', 'MAE'], cv=5, verbose=True)
The metrics used here are Root Mean Squared Error (RMSE) and Mean Absolute Error (MAE). These metrics give us an idea of how much our predictions deviate, on average, from the actual ratings in the dataset.
Once you run the code, you'll get an output with the test scores, which include the RMSE and MAE for each of the 5-fold cross-validation, as well as their average and the total time of computation.
Step 5: Making Predictions
With your model trained and evaluated, you can now make predictions for specific users and items.
uid = str(196) # raw user id
iid = str(302) # raw item id
# get a prediction for specific users and items.
pred = algo.predict(uid, iid, r_ui=4, verbose=True)
In the above example, the user with ID 196 and the item with ID 302 are used for the prediction. 'r_ui' is the true rating. 'verbose = True' means the function will print its results.
And there you have it—a basic movie recommendation engine using Python and the Surprise library. This tutorial should serve as a launching pad for your exploration of recommendation engines using Python, as you delve deeper into more complex models, different algorithms, and larger datasets. The opportunities for refinement and adjustment are vast, ensuring that your recommendation engine can evolve as your understanding and requirements grow.
Improving your recommendation engine: Tips and best practices
Building a basic recommendation engine is certainly a notable achievement. However, if you wish to take your system to the next level and enhance its performance, it's essential to employ a range of strategies, techniques, and best practices.
1. Expand your Dataset: One surefire way to improve your recommendation engine is by expanding your dataset. More data equals more information for your system to learn from. Consider incorporating additional user behaviors and item attributes, or even consider merging multiple datasets. This provides richer insight and can significantly improve recommendation quality.
2. Experiment with Different Algorithms: As we've discussed earlier, there's a variety of machine learning algorithms that can be used in recommendation engines. Trying different ones and comparing their performance can help identify the algorithm that works best for your specific scenario. Don't be afraid to experiment with different collaborative and content-based filtering methods, or even hybrid approaches.
3. Address the Cold Start Problem: The cold start problem - the challenge of making accurate recommendations for new users or items that have little to no interaction data - is a common hurdle in recommendation systems. One strategy to mitigate this is to use content-based filtering for new users or items, utilizing the information available about the user or the item to provide initial recommendations. For instance, for a new user, you could use demographic information or explicit feedback (like a short survey) to generate initial recommendations.
4. Implement a Popularity Filter: While personalized recommendations are the end goal, sometimes, popular items can be a safe bet, particularly for new users. Implementing a popularity filter, which suggests items that are currently trending or most-rated, could be a helpful supplement to your recommendation system.
5. Consider the Context: User preferences can be dependent on certain contexts such as time, location, or mood. By incorporating context into your recommendation engine, you can make your suggestions more relevant and timely. A user might prefer watching different genres of movies in the morning versus late at night, for example. Understanding and incorporating such context-dependent preferences can significantly improve your recommendations.
6. Constantly Evaluate and Update the model: Regularly evaluating your model with different metrics and updating it based on the latest user-item interactions can help keep your recommendations fresh and relevant. It's also worth regularly retraining your model as new data comes in, to ensure that it learns from the most recent user behaviors.
7. Personalize Recommendations Further: There are ways to go beyond the basic collaborative and content-based filtering techniques for more personalized recommendations. One such method is using Deep Learning algorithms. Deep Learning can discover intricate structures within the data and model complicated non-linear relationships. This can be particularly useful for large-scale and complex recommendation tasks.
Remember, the goal of a recommendation system is not only to predict user preferences accurately but also to increase user satisfaction, diversity, and the novelty of recommendations. Balancing accuracy with these other factors is key to building successful recommendation systems. While Python and its vast selection of libraries significantly simplify the development process, continuous improvement and fine-tuning of your model based on feedback and performance will go a long way in improving the reliability of your recommendations and the satisfaction of your users.
Summary
In conclusion, recommendation engines are powerful tools in today's digital landscape, assisting in providing a personalized user experience and elevating customer engagement. Python, with its easy-to-use syntax and rich collection of libraries and tools, is an excellent language for building these systems.
This comprehensive guide explores the fundamental concepts of recommendation systems, highlights the different machine learning algorithms used, and walks you through the process of building and refining a basic recommendation engine using Python. We've also discussed several strategies to enhance the performance of your recommendation systems, including expanding your dataset, experimenting with different algorithms, addressing the cold start problem, adding a popularity filter, considering user context, and continuously evaluating and updating your model.
The journey doesn't stop here. The field of recommendation engines is continuously evolving, and as a Python developer, data scientist, or researcher, there are always new techniques, tools, and methods to explore and implement. Continue to learn, experiment, and evolve your knowledge and skills to build robust, efficient, and effective recommendation engines that truly enhance user experiences.